Adding Sitemap to Next Js App Router
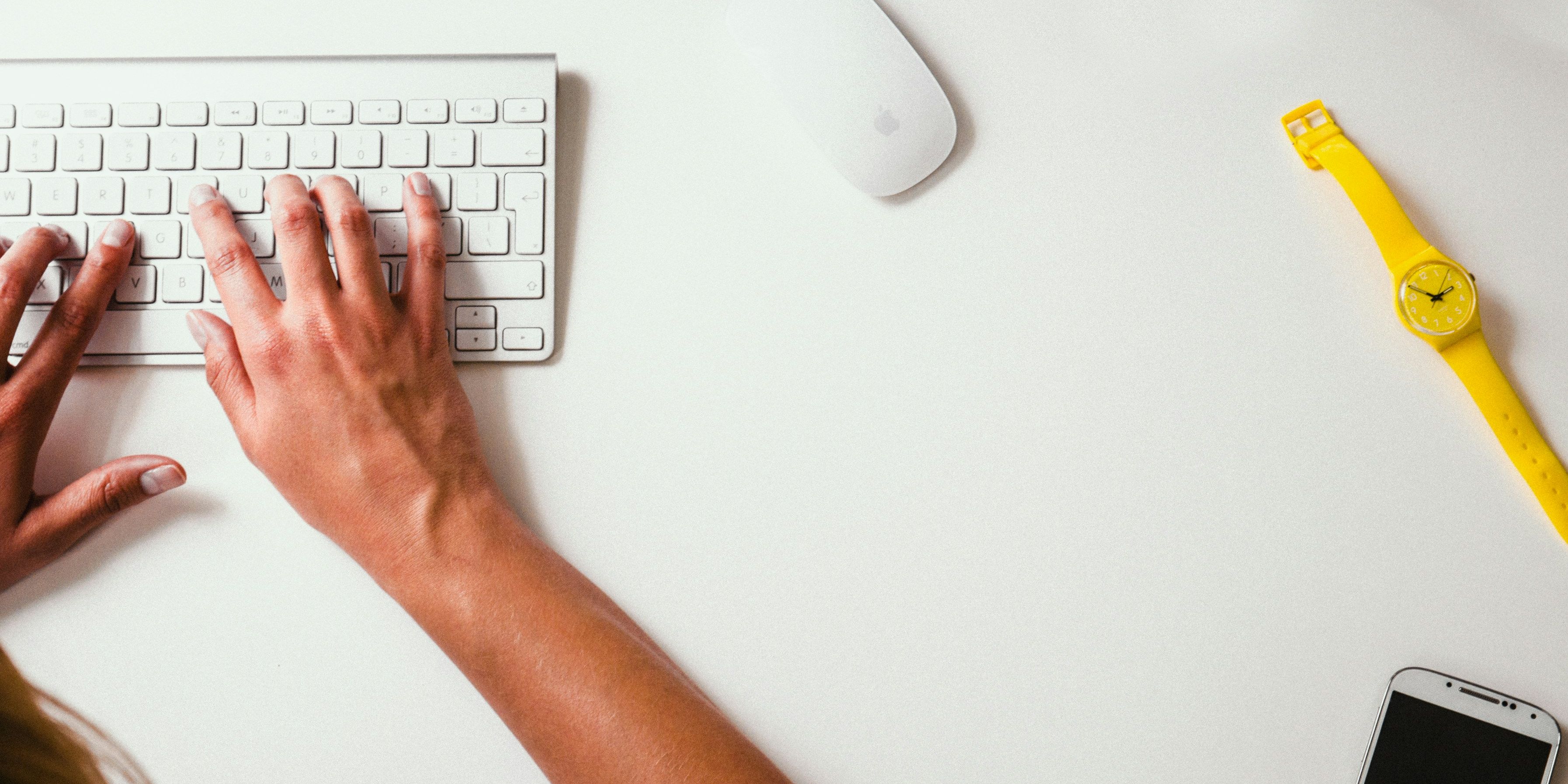
Introduction
You've built a website using Next.js App Router and want to appear on Google? While Google can often find and crawl your site links automatically, it's possible that your links are scattered and need a little help.
That's why you need to create a sitemap.xml file for your website.
What is sitemap.xml?
Copied from google documentation [Google doc]:
A sitemap is a file where you provide information about the pages, videos, and other files on your site, and the relationships between them. Search engines like Google read this file to crawl your site more efficiently. A sitemap tells search engines which pages and files you think are important in your site, and also provides valuable information about these files. For example, when the page was last updated and any alternate language versions of the page.
How do I create a sitemap?
Step one:
in app directory create a file called
in app directory create a file called
sitemap.ts

vs code screenshot
Step Two:
You need to return
MetadataRoute.Sitemap
which is an array that can be imported from Next like this import { MetadataRoute } from "next";
tsx
export default async function sitemap(): Promise<MetadataRoute.Sitemap> {
const postUrls = await getPostUrls();
return createSitemap([...defaultUrls, ...postUrls]);
}
Here there is three things we need to create:
1. getPostUrls();
2. createSitemap;
3. defaultUrls;
lets start with defaultUrls which will literally be your default urls. That means home, about, contact etc...
Anything you want can be added to default url.
Anything you want can be added to default url.
For my default urls I like to create a type called Link:
ts
type Link = {
url: string;
lastModified?: Date;
changeFrequency?:
| "always"
| "hourly"
| "daily"
| "weekly"
| "monthly"
| "yearly"
| "never";
priority: number;
}
const POST_BASE_PATH = "posts"
const defaultUrls: Link[] = [
{
url: "",
changeFrequency: "daily",
priority: 1,
lastModified: new Date(),
},
{ url: POST_BASE_PATH, priority: 1 },
];
Then createSitemap concatenating with baseUrl and generate the full route.
ts
const createSitemap = (locations: Link[]): MetadataRoute.Sitemap => {
const baseUrl = process?.env?.NEXT_PUBLIC_URL;
return locations.map((location) => {
return {
url: `${baseUrl}/${location.url}`,
lastModified: location.lastModified,
changeFrequency: location.changeFrequency,
priority: location.priority,
};
});
};
finally getPostUrls() is to get dynamic urls where you can call your api to get the slugs for all the posts and create routes for them.
Finally:
you should now be able to go to "your-website/sitemap.xml" to see the map. checkout my sitemap at https://georgeblog.tech/sitemap.xml.
Full code image for reference:

full code image
Thank you!